FastAPI§
To run apps built with the FastAPI web framework using Unit:
Install Unit with a Python 3.6+ language module.
Create a virtual environment to install FastAPI’s PIP package:
$ cd /path/to/app/ $ python3 --version Python 3.Y.Z $ python3 -m venv venv $ source venv/bin/activate $ pip install fastapi $ deactivate
Warning
Create your virtual environment with a Python version that matches the language module from Step 1 up to the minor number (3.Y in this example). Also, the app type in Step 5 must resolve to a similarly matching version; Unit doesn’t infer it from the environment.
Let’s try a version of a tutorial app, saving it as /path/to/app/asgi.py:
from fastapi import FastAPI app = FastAPI() @app.get("/") async def root(): return {"message": "Hello, World!"}
Note
For something more true-to-life, try the RealWorld example app; just install all its dependencies in the same virtual environment where you’ve installed FastAPI and add the app’s environment variables like DB_CONNECTION or SECRET_KEY directly to the app configuration in Unit instead of the .env file.
Run the following command (as root) so Unit can access the application directory:
# chown -R unit:unit /path/to/app/
Note
The unit:unit user-group pair is available only with official packages, Docker images, and some third-party repos. Otherwise, account names may differ; run the ps aux | grep unitd command to be sure.
For further details, including permissions, see the security checklist.
Next, prepare the FastAPI configuration for Unit (use real values for type, home, and path):
{ "listeners": { "*:80": { "pass": "applications/fastapi" } }, "applications": { "fastapi": { "type": "python 3.Y", "path": "/path/to/app/", "home": "/path/to/app/venv/", "module": "asgi", "callable": "app" } } }
Upload the updated configuration. Assuming the JSON above was added to
config.json
. Run the following command as root:# curl -X PUT --data-binary @config.json --unix-socket \ /path/to/control.unit.sock http://localhost/config/
Note
The control socket path may vary; run unitd -h or see Startup and Shutdown for details.
After a successful update, your app should be available on the listener’s IP address and port:
$ curl http://localhost Hello, World!
Alternatively, try FastAPI’s nifty self-documenting features:
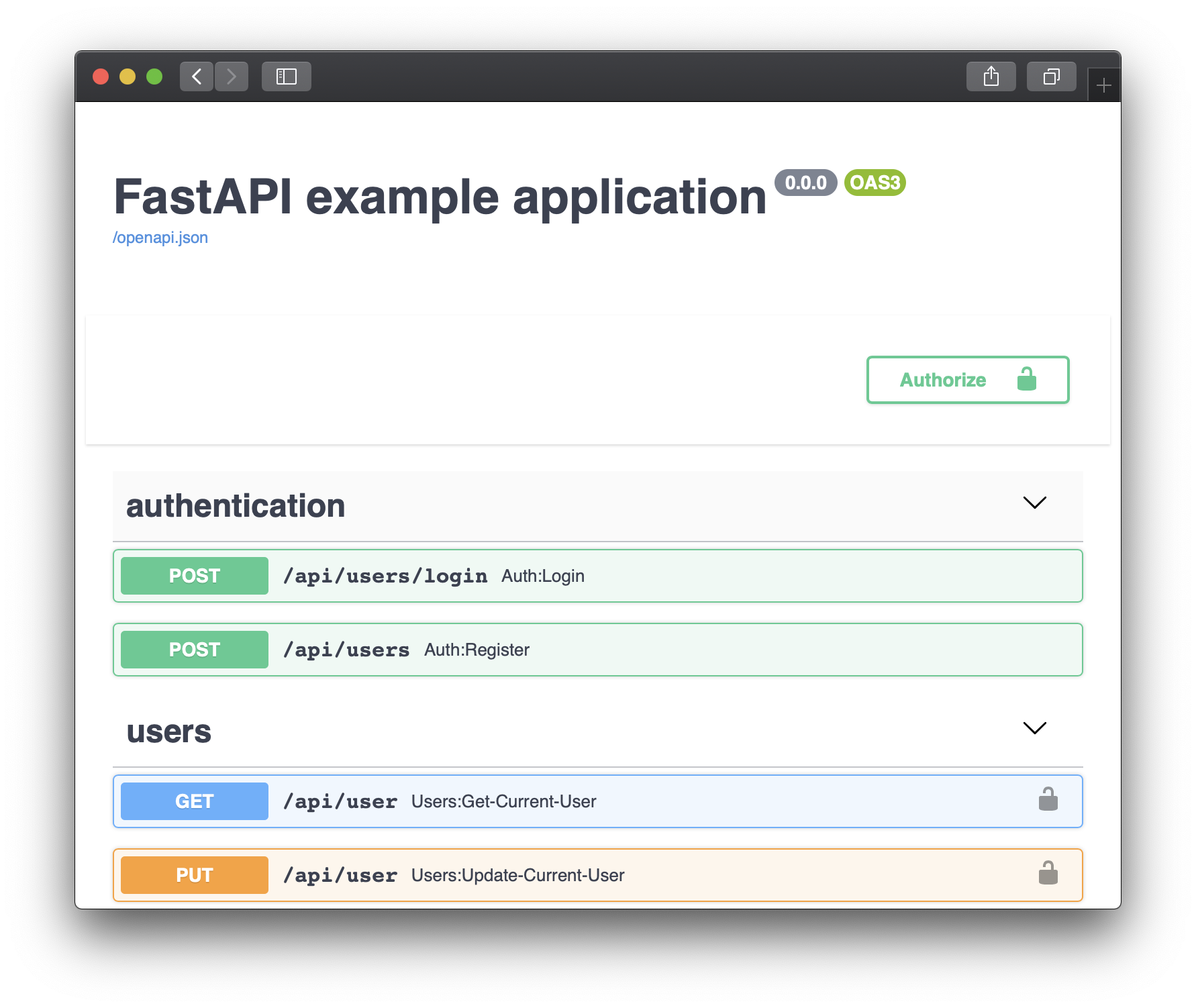